반응형
Design Patterns - Decorator Pattern
(원문 위치 : http://www.tutorialspoint.com/design_pattern/decorator_pattern.htm )
데코레이션 패선은 사용자가 구조를 대체하는 것 없이 이미 존재하는 객체에 새로운 기능성을 추가하는 것을 허락한다. 이 디자인 패턴의 형태는 이 패턴이 이미 존재하는 클래스에 포장하는 것처럼 동작하기 때문에 구조적 패턴에 포함된다.
이 패턴은 원본 객체를 포장하는 데코레이션 객체를 생성하고 온전한 class methods signature를 유지하면서 부가적인 기능을 제공한다.
여기서는 shape 클래스를 대체하는 것 없이 몇몇 색을 갖는 shape를 꾸밀(decorate) 아래 예제를 통해 데코레이션 패턴의 용도를 보여줄 것이다.
Implementation
Shape 인터페이스와 Shape 인터페이스를 구현하는 구체적인 클래스를 생성할 것이다. 그리고 Shape 인터페이스를 구현하고 인스턴스 변수(instance variable)로써 Shape 객체를 갖는 추상 decorator클래스인 ShapeDecorator를 생성할 것이다.
RedShapeDecorator는 ShapeDecorator를 구현하는 구체적 클래스이다.
DecoratorPatternDemo, demo 클래스는 Shape객체를 꾸미기 위해 RedShapeDecorator를 사용할 것이다.
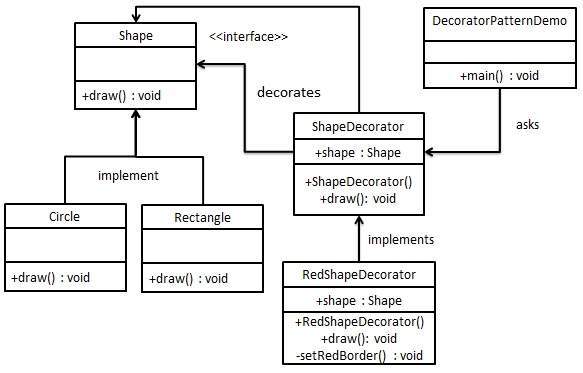
Step 1
인터페이스를 생성한다.
Shape.java
public interface Shape { void draw(); }
Step 2
동일 인터페이스를 구현하는 구체적인 클래스를 생성한다.
Rectangle.java
public class Rectangle implements Shape { @Override public void draw() { System.out.println("Shape: Rectangle"); } }
Circle.java
public class Circle implements Shape { @Override public void draw() { System.out.println("Shape: Circle"); } }
Step 3
Shape 인터페이스를 구현하는 추상 decorator클래스를 생성한다.
ShapeDecorator.java
public abstract class ShapeDecorator implements Shape { protected Shape decoratedShape; public ShapeDecorator(Shape decoratedShape){ this.decoratedShape = decoratedShape; } public void draw(){ decoratedShape.draw(); } }
Step 4
ShapeDecorator 클래스를 확장하는 구체적인 decorator 클래스를 생성한다.
RedShapeDecorator.java
public class RedShapeDecorator extends ShapeDecorator { public RedShapeDecorator(Shape decoratedShape) { super(decoratedShape); } @Override public void draw() { decoratedShape.draw(); setRedBorder(decoratedShape); } private void setRedBorder(Shape decoratedShape){ System.out.println("Border Color: Red"); } }
Step 5
Shape 객체를 꾸미기 위해 RedShapeDecorator를 사용한다.
DecoratorPatternDemo.java
public class DecoratorPatternDemo { public static void main(String[] args) { Shape circle = new Circle(); Shape redCircle = new RedShapeDecorator(new Circle()); Shape redRectangle = new RedShapeDecorator(new Rectangle()); System.out.println("Circle with normal border"); circle.draw(); System.out.println("\nCircle of red border"); redCircle.draw(); System.out.println("\nRectangle of red border"); redRectangle.draw(); } }
Step 6
결과를 확인한다.
Circle with normal border Shape: Circle Circle of red border Shape: Circle Border Color: Red Rectangle of red border Shape: Rectangle Border Color: Red
반응형