반응형
Design Pattern - Abstract Factory Pattern
(원문 위치 : http://www.tutorialspoint.com/design_pattern/abstract_factory_pattern.htm )
추상 팩토리 패턴은 다른 팩토리들을 생성하는 수퍼 팩토리를 피하며 일한다. 이 팩토리는 팩토리의 팩토리로써 불리어진다. 이 디자인 패턴 형태는 이 패턴이 객체를 생성하는 좋은 방법중 하나를 제공하므로 생성적 패턴에 포함된다.
추상 팩토리 패턴에서 인터페이스는 명시적으로 클래스를 구체화하는 것 없이 객체와 관련된 팩토리를 생성할 책임을 갖는다. 생성된 각 팩토리는 팩토리 패턴에 따라 객체를 줄 수 있다.
Implementation
Shape와 Color 인터페이스를 생성하고 이 인터페이스를 구현하는 클래스를 구체화한다. 다음단계로 추상 팩토리 클래스 AbstractFactory를 생성한다. 팩토리 클래스 ShapeFactory와 ColorFactory는 AbstractFactory를 확장한 각 팩토리에 정의된다. 팩토리 creator/generator 클래스 FactoryProducer는 생성되어 진다.
AbstractFactoryPatternDemo, 데모 클래스는 AbstractFactory객체를 얻기위해 FactoryProducer를 사용한다. 이것은 필요한 객체의 타입을 얻기위해 AbstractFactory에 정보(모양을 위한 CIRCLE/RECTANGLE/SQUARE)를 전달할 것이다. 또한 필요한 객체의 타입을 얻기위해 AbstractFactory에 정보(색을 위한 RED/GREEN/BLUE)를 전달한다.
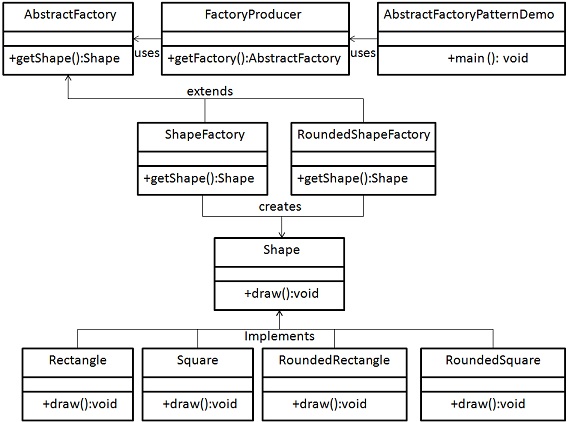
Step 1
Shapes를 위한 인터페이스를 생성한다.
Shape.java
public interface Shape { void draw(); }
Step 2
동일 인터페이스를 구현하는 구체적인 클래스를 생성한다.
Rectangle.java
public class Rectangle implements Shape { @Override public void draw() { System.out.println("Inside Rectangle::draw() method."); } }
Square.java
public class Square implements Shape { @Override public void draw() { System.out.println("Inside Square::draw() method."); } }
Circle.java
public class Circle implements Shape { @Override public void draw() { System.out.println("Inside Circle::draw() method."); } }
Step 3
Colors를 위한 인터페이스를 생성한다.
Color.java
public interface Color { void fill(); }
Step4
동일 인텊이스를 구현하는 구체적인 클래스를 생성한다.
Red.java
public class Red implements Color { @Override public void fill() { System.out.println("Inside Red::fill() method."); } }
Green.java
public class Green implements Color { @Override public void fill() { System.out.println("Inside Green::fill() method."); } }
Blue.java
public class Blue implements Color { @Override public void fill() { System.out.println("Inside Blue::fill() method."); } }
Step 5
Color와 Shape 객체를 위한 팩토리를 얻기 위한 추상 클래스를 생성한다.
AbstractFactory.java
public abstract class AbstractFactory { abstract Color getColor(String color); abstract Shape getShape(String shape) ; }
Step 6
주어진 정보를 바탕으로 구체적인 클래스의 객체를 생성하기 위한 AbstractFactory를 확장하는 팩토리 클래스를 생성한다.
ShapeFactory.java
public class ShapeFactory extends AbstractFactory { @Override public Shape getShape(String shapeType){ if(shapeType == null){ return null; } if(shapeType.equalsIgnoreCase("CIRCLE")){ return new Circle(); }else if(shapeType.equalsIgnoreCase("RECTANGLE")){ return new Rectangle(); }else if(shapeType.equalsIgnoreCase("SQUARE")){ return new Square(); } return null; } @Override Color getColor(String color) { return null; } }
ColorFactory.java
public class ColorFactory extends AbstractFactory { @Override public Shape getShape(String shapeType){ return null; } @Override Color getColor(String color) { if(color == null){ return null; } if(color.equalsIgnoreCase("RED")){ return new Red(); }else if(color.equalsIgnoreCase("GREEN")){ return new Green(); }else if(color.equalsIgnoreCase("BLUE")){ return new Blue(); } return null; } }
Step 7
Shape 또는 Color같은 정보를 전달하는 것으로 팩토리를 얻기 위한 팩토리 generator/producer 클래스를 생성한다.
public class FactoryProducer { public static AbstractFactory getFactory(String choice){ if(choice.equalsIgnoreCase("SHAPE")){ return new ShapeFactory(); }else if(choice.equalsIgnoreCase("COLOR")){ return new ColorFactory(); } return null; } }
Step 8
type같은 정로블 전달하는 것으로 구체적인 클래스의 팩토리를 얻기 위한 AbstractFactory를 얻는 FactoryProducer를 사용한다.
AbstractFactoryPatternDemo.java
public class AbstractFactoryPatternDemo { public static void main(String[] args) { //get shape factory AbstractFactory shapeFactory = FactoryProducer.getFactory("SHAPE"); //get an object of Shape Circle Shape shape1 = shapeFactory.getShape("CIRCLE"); //call draw method of Shape Circle shape1.draw(); //get an object of Shape Rectangle Shape shape2 = shapeFactory.getShape("RECTANGLE"); //call draw method of Shape Rectangle shape2.draw(); //get an object of Shape Square Shape shape3 = shapeFactory.getShape("SQUARE"); //call draw method of Shape Square shape3.draw(); //get color factory AbstractFactory colorFactory = FactoryProducer.getFactory("COLOR"); //get an object of Color Red Color color1 = colorFactory.getColor("RED"); //call fill method of Red color1.fill(); //get an object of Color Green Color color2 = colorFactory.getColor("Green"); //call fill method of Green color2.fill(); //get an object of Color Blue Color color3 = colorFactory.getColor("BLUE"); //call fill method of Color Blue color3.fill(); } }
Step 9
결과를 확인한다.
Inside Circle::draw() method. Inside Rectangle::draw() method. Inside Square::draw() method. Inside Red::fill() method. Inside Green::fill() method. Inside Blue::fill() method.
반응형